Server Side Library
Introduction
The CoolCalc API is designed as a server-to-server web service. In OEM and distributor installations a small server-side library is implemented on your servers as part of the CoolCalc integration.
The principal responsibilities of the server-side library are:
- Provide user information for the current session to the CoolCalc JavaScript library.
- Forward HTTP requests from the browser to the CoolCalc API (see section on data privacy)
- Implement PDF conversion and MJ8 report email functionality.
The coolcalc-clients repository on Github (https://github.com/silverliningco/coolcalc-clients) contains sample implementations in various languages. Follow the instructions in this chapter and the README files for your specific language/platform for configuration and implementation details.
The server-side libraries can be easily ported to any modern language or framework. If your application runs on a language or platform not available in our Github repository please contact our development team.
Implementation required on your side:
- Download/install coolcalc-client library for your specific language or platform.
- Enter your organization's API credentials in the sample library.
The README files will indicate location of the API credentials in each version of the libraries.
Data privacy
In the CoolCalc data store all data is segregated by API customer and additionally by the unique id(s) for the end user, which are provided in the REST URL. The CoolCalc API never sees identifiable end user information such as names or email addresses.
The server-side library implements 2 essential responsibilities related to data privacy:
- Provide the API customer's credentials (ie. your organization's credentials) in all requests to the CoolCalc API.
- Verify the end user's unique id(s) in the REST URL in all requests to the CoolCalc API.
User interaction in the CoolCalc user interface triggers XHR requests from the browser to your local server, by means of the CoolCalc JavaScript library. The server-side library effectively forwards the XHR requests from the browser to the CoolCalc API and forwards the response of the CoolCalc API to the browser. For example:
- Browser requests URL on your local server:
- The server-side library repeats the request to:
- The server-side library forwards the response from the CoolCalc API to the browser.
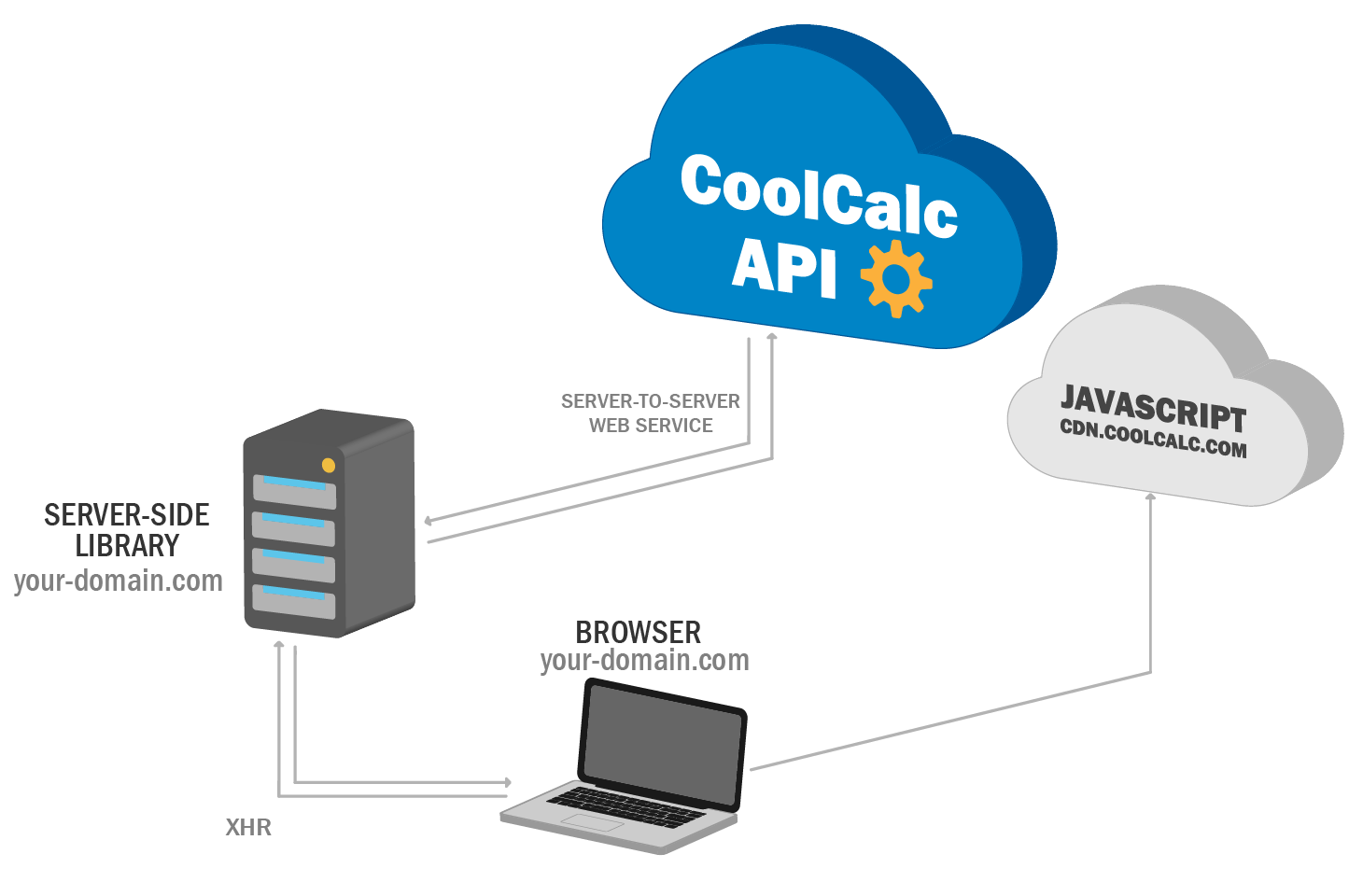
Your server as the identity provider knows and authenticates its end users. The contract between your server and the CoolCalc API is simply that the user identification provided in the REST URL is valid. For example, in the sample API request below your server is guaranteeing to the CoolCalc API that the end user account nr is "12345":
After the initial URL segment that contains your customer account nr. the CoolCalc API verifies foreign keys for all subsequent URL segments to prevent Insecure Direct Object References (IDOR).
Why not CORS?
A frequent question from API customers and developers is why does CoolCalc require a server-side library and why not use CORS?
The reason is simple: CORS is typically used to do the opposite of what the CoolCalc API does. With CORS, any site (your-domain.com) can make credentialed requests to a different domain, which is typically an identity provider. If all end users of our various API customers were logged in on a CoolCalc domain the browser could use CORS to make credentialed requests from your-domain.com to CoolCalc. However, that is not how CoolCalc is designed. End users are known to and authenticated by our various API clients (your-domain.com) but entirely unknown to the CoolCalc API other than by the unique IDs that your-domain.com provides for each end user.
The above is not a programming constraint but a key part of the real-world, commercial value of CoolCalc. OEMs and distributors integrate with CoolCalc to add value to the relationship with their own customers. The end users enjoy the benefits of the CoolCalc applications without being a direct or first-degree CoolCalc customer.
Local endpoints
In a typical installation the local server-side library exposes the following endpoints:
- A user identification endpoint to provide end user identification for the current session to the CoolCalc JavaScript library.
- The main “CoolCalc client” endpoint that forwards XHR requests from the browser to the CoolCalc API.
- A dedicated “MJ8 report” endpoint that forwards only XHR requests for the MJ8 report resource to the CoolCalc API.
- A special purpose endpoint for HTML to PDF conversion.
- A special purpose endpoint to email MJ8 reports to users.
Each of the endpoints is documented in the following sections. The sample client side libraries in our Github repository include boilerplate code for these endpoints but in most cases some internal details will need to be implemented on your side because the specifics of session and user management may vary in each client's existing code base.
Optionally, if you want to implement local or custom REST resources above and beyond the REST resources provided by the CoolCalc API, your local server-side library needs to implement an additional endpoint that provides the static HTML for those resources to the CoolCalc JavaScript library. See the section on customizing CoolCalc for further details.
User identification endpoint
The CoolCalc user interface normally opens on the user's project list. As part of the user interface initialization, the CoolCalc JavaScript library queries a local endpoint on your server for the end user's identification. With the end user's identification, the JavaScript library constructs the entry URL for the user's project list and then makes the initial API call.
From the web root, the typical location for the end user identification endpoint is:
- ASP: /coolcalc/user/{version}/session-user.ashx
- Java: /coolcalc/user/{version}/SessionUser
- PHP: /coolcalc/user/{version}/session-user.php
The output of the end user identification endpoint must be a JSON string as follows:
{ “userReference”: “12345”, “dealerReference”: “xyz-345-abc”, “isAdmin”: true }
If the boolean isAdmin is true the user will have access to all projects of all individual end users in their organization. If isAdmin is false, the user only has access to their own projects.
Implementation required on your side:
- Implement the JSON output of the user identification endpoint based on your existing session management and user identification mechanisms
- If you allow unauthenticated users to use your CoolCalc installation, the end user endpoint must assign unauthenticated users a random/unique “John or Jane Doe” id for the duration of the session.
Check the README files in our sample client side for implementation details of your specific language or platform. If you expose your CoolCalc applications to unauthenticated users see the section on customizing CoolCalc for additional details on “John or Jane Doe” ids.
CoolCalc client endpoint
As explained in the data privacy section, one of the principal responsibilities of the server side library is forwarding the XHR requests from the browser to the CoolCalc API. A single endpoint on your local server is used to forward all browser requests for a given entry point at the CoolCalc API. Currently, all requests except for direct requests to an MJ8 report resource are based on the “/dealers” entry point.
From the web root, the typical location for “dealers” endpoint is:
- ASP: /coolcalc/client/{version}/dealers.ashx
- Java: /coolcalc/client/{version}/dealers
- PHP: /coolcalc/client/{version}/dealers.php
When initializing the user interface, the CoolCalc JavaScript library queries your end user identification endpoint to download the user's project list. The JavaScript library will make all subsequent API requests based on the entry URL that contains the user's identification. However, this is done in the client (browser) and nothing can be done to prevent a dishonest user from manually entering a URL with a different account nr in the browser, thereby downloading the project list for another user.
To avoid such an exploit, the server-side library must verify that the customer identification in the REST URL corresponds to the current session user before making any HTTP requests to the CoolCalc API.
Implementation required on your side:
- Check that the account nr in the REST URL corresponds to the actual end user based on your existing session management and user identification mechanisms. There are many REST URLs requested by the browser and the specifics of the URLs may change over time but the customer identification will always be a “.../dealers/{dealerId}/...” segment. The server-side library only needs to split the REST URL on the “/” character and check that the value of the segment following “dealers” corresponds to the customer identification (account nr) for the end user.
The README files in our sample client side code indicate where to implement the end user verification for your chosen language.
MJ8 report endpoint
In “the real world” it is often necessary to access an MJ8 report outside the normal flow of the CoolCalc user interface, for example, for PDF conversion, email or link sharing.
However, the design of the CoolCalc applications – specifically the data privacy mechanisms explained in this chapter – prevent sharing any resources from the user interface without a valid session. Put simply, nobody can access any resource in the CoolCalc UI without proving they are the owner of that resource.
To allow MJ8 report access without an existing session while providing an acceptable measure of data privacy, MJ8 reports are identified by unique, not guessable ids and the server-side library implements a dedicated endpoint for MJ8 report resources. This dedicated MJ8 report endpoint differs from the main CoolCalc client endpoint only in that it does not require a valid session and returns only the MJ8 report resource by unique ID.
From the web root, the typical location for the MJ8 report endpoint is:
- ASP: /coolcalc/client/{version}/mj8-reports.ashx
- Java: /coolcalc/client/{version}/MJ8Reports
- PHP: /coolcalc/client/{version}/mj8-reports.php
Your web server should map all requests based on a “/coolcalc/client/{version}/dealers/...” URL to this endpoint, regardless of the URL segments following the “/dealers” segment.
The MJ8 report endpoint is implemented in each of the sample client side libraries in our Github repository. In most cases no configuration or implementation is required on your side.
HTML to PDF endpoint
The static HTML for the downloadable MJ8 report is hosted on the client's server for the purposes of customization and branding. HTML to PDF conversion must be implemented on the client side.
The section on customizing CoolCalc provides a complete overview of the HTML to PDF implementation. In a typical installation, the server-side library implements a dedicated, special purpose endpoint to convert HTML to PDF. The CoolCalc JavaScript library automatically invokes this endpoint and provides the HTML URL to be converted in the body of a POST request.
Implementation required on your side:
- Implement the special purpose HTML to PDF endpoint on your local server.
- You can use any tool (eg. headless Chrome) or API of your choice to convert HTML to PDF.
- The HTML to PDF endpoint only needs to check the HTML URL provided by the caller (XHR request from the CoolCalc JavaScript library) and convert the given URL to PDF.
The README files in our sample client side code indicate where to implement the end user verification for your chosen language.
Email endpoint
As with PDF conversion, the email/share functionality of the MJ8 report must be implemented on the client side.
The section on customizing CoolCalc provides a complete overview of the email/share report implementation. In a typical installation, the server-side library implements a dedicated, special purpose endpoint to email the MJ8 report in PDF format. The CoolCalc JavaScript library automatically invokes this endpoint and provides the HTML URL of the report as well as the desired “email to” address in the body of a POST request.
Implementation required on your side:
- Implement the special purpose “email/share report” endpoint on your local server.
- The actual email send functionality can be implemented using any library or API of your choice.
- The HTML to PDF endpoint only needs to check the HTML URL and “email to” parameters provided by the caller (XHR request from the CoolCalc JavaScript library) then convert the given URL to PDF and email the PDF to the desired address.
Refer to the customizing CoolCalc section for a complete overview of the client-server interaction for the email/share report functionality.